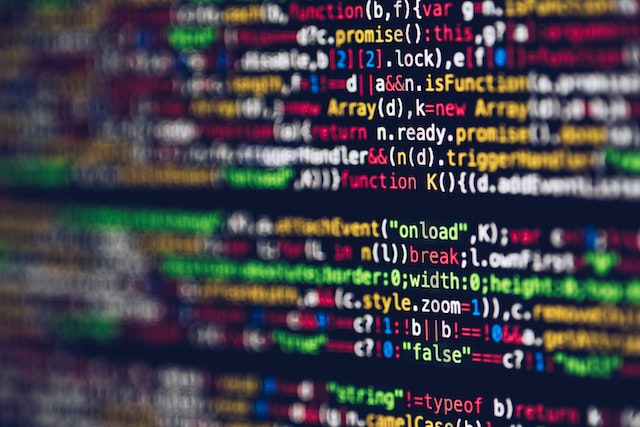
What is JavaScript array?
JavaScript Array is really important.
Arrays can enable storing a collection of multiple items under a single variable name. These data and data structures are stored in the form of sequence of memory locations. If one needs to access the array they can use the index number like 0,1,2,3 and can store data types like Integer, Float, String, and Boolean including all the primitive data types.
Objects in JavaScript
Objects in JavaScript arrays store a specific number of values in an order which is index based.
Objects represent a particular “thing” in the code, ity could be a car, a person, character in the game and they are good to use when one knows where the data is being processed. The objects are defined by a set of characteristics that consists of a key and a value.
The properties in the object can be accessed, added, changed, and removed by using either dot or bracket notation.
Array and Array of Objects of JavaScript
Whether to use array or array objects depends on the situation one is trying to address and therefore it makes sense to take a look at the properties of both array and array of objects.
Arrays:
- Best to use when the elements are numbers
- The data inside an array is called Element
- Elements can be manipulated using []
- pop() function can be used to pop out elements
- In array iterating is possible using For loop, For..in, For..of, and ForEach()
Array of Objects:
- Best to use when Elements strings
- Data inside objects are known as properties with a key and value
- The properties can be manipulated using both .DOT notation and []
- Delete keyword can be used to delete key or properties
- In array object iteration is possible using For..in, For..of, and ForEach()
If one has to store related data for a faster retrieval then JavaScript array is the perfect option.
And JavaScript array method cannot just save you time but also help you in efficient coding.
Let’s understand how.
The For Loop
If a block of code needs to be repeated, loops can be used to achieve that, say for example, a message that needs to show 100 times, loop can ensure that.
The for loop is a structure for control flow and goes through the values of an array systematically, one by one. The duration or the distance of the loop is determined by conditions.
For an efficient loop, the conditions need to be defined adequately.
The syntax of the for loop is:
for (initialExpression; condition; updateExpression) {
// for loop body
}
- The initial expression initiates/declares variable and executes just once
- The condition is evaluated
- If the condition is false the loop is terminated
- If the condition is true the block code in the for loop is executed
- The update expression can update the value of Initial Expression but only when the condition is true
- The condition is evaluated again and continues until the condition is false
sort() Method –
JavaScript has an inbuilt method sort() that can arrange an array of alphabets in lexicographical order.
Syntax: sort() Method
Arrays.sort()
It considers three parameters :
- The array to be sorted
- The from_index to be sorted
- The index of the last element, exclusive, to be sorted (Referred to as last_index)
Let’s try arranging an array of alphabets:
array = [‘r’, ‘p’, ‘s’, ‘q’] ;
array.sort() ;
console.log(array) ; // [ ‘p’, ‘q’, ‘r’, ‘s’ ]
One can also sort numbers in ascending or descending order using this.
reverse() Method
As the name suggests it can reverse the arrangement order of items in an array.
let array = [‘p’,’q’,’r’,’s’];
array.reverse();
console.log(array);
// [ ‘s’, ‘r’, ‘q’, ‘p’ ]
toString() Method
This method can transform an array into a single string.
const vegetables = [“Potatoes”, “Onions”, “Broccoli”, “Peas”];
let text = vegetables.toString();
console.log(text);
//Potatoes,Onions,Broccoli,Peas
The output is in the form of one string with array values separated by comma.
Javascript array Object Methods
Two methods of JavaScript array objects are orEach() and reduce() methods
The forEach method
let string = ‘’;
const fruits = [“po”, “ta”, “toe”];
Vegetables.forEach((vegetable)=>{
string+=vegetable
});
console.log(string);
//potatoe
The reduce() method helps to reduce an array to a single value as follows :
const numbers = [150, 50, 25];
let result = numbers.reduce(myFunc);
function myFunc(total, num) {
return total + num;
}
console.log(result) ;
//225
Conclusion
Arrays can be used effectively by using the above methods and the most suitable ones can be chosen to meet the needs of specific situations.